Some of the cases we need to create an image dynamically when the web page is loading, Then a question raised in the mind - Can I convert text to image in PHP? the answer is Yes! why not. For doing this activity you just need to do a few steps. These steps are given below:
- Check your GD library extension is on in your current PHP version.
- If it does not enable simply just un-comment it.
- Include phpTextToImage class in your project.
- Create an object of this class.
- Call create image function with your text.
- Call save function with your file name and location.
This library has numbers of features which fulfill your all requirement, these features are given:
- Create dynamically text image.
- Set your text font.
- Choose an image background.
- Set your text color also.
- Adjust your image height and width.
Let's start with the example. First of all, include the phpTextToImage class in your project files.
<?php
/**
* phpTextToImage class
* This class converts text to image in PHP
*
* @author Legend Blogs
* @link https://www.legendblogs.com
*/
class phpTextToImage {
private $image;
/**
* Create image from text
* @param string text to convert into image
* @param string textColor
* @param string backgroundColor
* @param int font size of text
* @param int width of the image
* @param int height of the image
*/
function createImage($text, $textColor = '', $backgroundColor = '', $fontSize = 22, $imgWidth = 600, $imgHeight = 300) {
//text font path
$font = 'font/Pacifico-Regular.ttf';
//create the image
$this->image = imagecreatetruecolor($imgWidth, $imgHeight);
$colorCode = array('#ffffff','#db3236', '#f4c20d', '#3cba54', '#4c53cc', '#56aad8', '#61c4a8');
if ($backgroundColor == '') {
/* select random color */
$backgroundColor = $this->hexToRGB($colorCode[rand(0, count($colorCode) - 1)]);
} else {
/* select background color as provided */
$backgroundColor = $this->hexToRGB($backgroundColor);
}
if ($textColor == '') {
/* select random color */
$textColor = $this->hexToRGB($colorCode[rand(0, count($colorCode) - 1)]);
} else {
/* select background color as provided */
$textColor = $this->hexToRGB($colorCode[rand(0, count($textColor) - 1)]);
}
$textColor = imagecolorallocate($this->image, $textColor['r'], $textColor['g'], $textColor['b']);
$backgroundColor = imagecolorallocate($this->image, $backgroundColor['r'], $backgroundColor['g'], $backgroundColor['b']);
imagefilledrectangle($this->image, 0, 0, $imgWidth - 1, $imgHeight - 1, $backgroundColor);
//break lines
$splitText = explode("\\n", $text);
$lines = count($splitText);
$angle = 0;
foreach ($splitText as $txt) {
$textBox = imagettfbbox($fontSize, $angle, $font, $txt);
$textWidth = abs(max($textBox[2], $textBox[4]));
$textHeight = abs(max($textBox[5], $textBox[7]));
$x = (imagesx($this->image) - $textWidth) / 2;
$y = ((imagesy($this->image) + $textHeight) / 2) - ($lines - 2) * $textHeight;
$lines = $lines - 1;
//add the text
imagettftext($this->image, $fontSize, $angle, $x, $y, $textColor, $font, $txt);
}
return true;
}
/* function to convert hex value to rgb array */
protected function hexToRGB($colour) {
if ($colour[0] == '#') {
$colour = substr($colour, 1);
}
if (strlen($colour) == 6) {
list( $r, $g, $b ) = array($colour[0] . $colour[1], $colour[2] . $colour[3], $colour[4] . $colour[5]);
} elseif (strlen($colour) == 3) {
list( $r, $g, $b ) = array($colour[0] . $colour[0], $colour[1] . $colour[1], $colour[2] . $colour[2]);
} else {
return false;
}
$r = hexdec($r);
$g = hexdec($g);
$b = hexdec($b);
return array('r' => $r, 'g' => $g, 'b' => $b);
}
/**
* Display image
*/
function showImage() {
header('Content-Type: image/png');
return imagepng($this->image);
}
/**
* Save image as png format
* @param string file name to save
* @param string location to save image file
*/
function saveAsPng($fileName = 'text-image', $location = '') {
$fileName = $fileName . ".png";
$fileName = !empty($location) ? $location . $fileName : $fileName;
return imagepng($this->image, $fileName);
}
/**
* Save image as jpg format
* @param string file name to save
* @param string location to save image file
*/
function saveAsJpg($fileName = 'text-image', $location = '') {
$fileName = $fileName . ".jpg";
$fileName = !empty($location) ? $location . $fileName : $fileName;
return imagejpeg($this->image, $fileName);
}
}
Now, create your project file where you want to create the image of your text dynamically, and just include class and create an object like this:
//include phpTextToImage class
require_once 'phpTextToImage.php';
//create img object
$img = new phpTextToImage;
After that, simply use this $img object to create your image with createImage function:
$text = 'Welcome to Legend Blogs.\nConvert Text To Image In PHP.';
$img->createImage($text);
If you want to save the image in your physical location you just need to call a saveAsPng() or saveAsJpg() function with your filename and location:
$fileName = "legendblogs";
$img->saveAsPng($fileName);
Your final code is:
<!DOCTYPE html>
<?php
//include phpTextToImage class
require_once 'phpTextToImage.php';
//create img object
$img = new phpTextToImage;
?>
<html lang="en">
<head>
<title>Convert Text To Image In PHP</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script src="//maxcdn.bootstrapcdn.com/bootstrap/3.2.0/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.3.1/css/all.css" crossorigin="anonymous">
</head>
<body>
<div class="container">
<h2 class="text-danger">Convert Text To Image In PHP</h2>
<h4>Refresh Your Page To Change Color</h4>
<?php
//create image from text
$text = 'Welcome to Legend Blogs.\nConvert Text To Image In PHP.';
$img->createImage($text);
//display image
$fileName = "legendblogs";
$img->saveAsPng($fileName);
?>
<img src="<?php echo $fileName; ?>.png" />
</div>
</body>
</html>
The output of the given text:
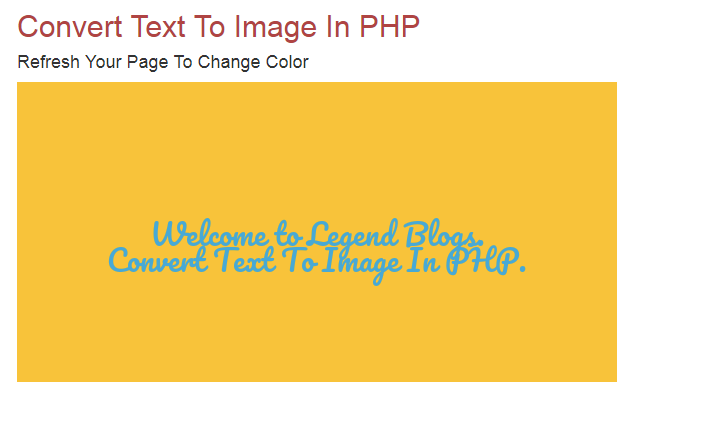
(1) Comments
Braulio
Hi, bro! thanks it is working fine!
How can I use an array of many images?
Thanks.
Write a comment